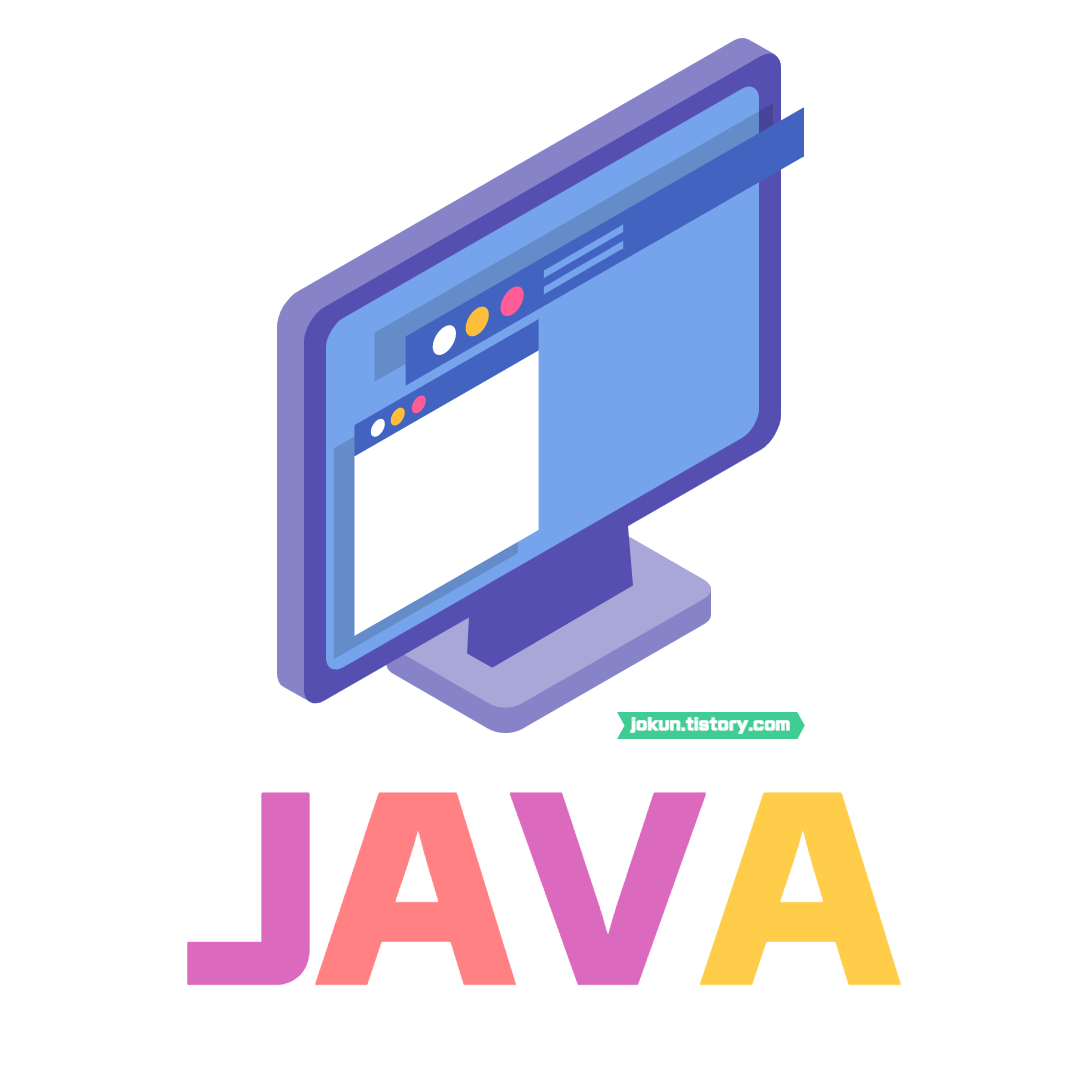
문제은행[2]
📑문제
1. 65 ~ 122 사이의 랜덤한 문자를 생성하도록 한다.
여기서 소문자나 대문자가 아니라면 다시 생성하도록 프로그램을 만들어보자
2. 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ... 일명 피보나치 수열의 20번째 항을 구하도록 프로그램 해보자!
3. 1, 1, 1, 2, 3, 4, 6, 9, 13, 19, 28, 41, 60, 88, 129, ...
이와 같은 숫자의 규칙을 찾아 25번째 항을 구하도록 프로그램 해보자!
4. 구구단 7단을 출력해보자!
5. 1 ~ 100까지 숫자중 짝수만 출력해보자.
6. 1 ~ 100까지 숫자중 3의 배수만 출력해보자!
7. 1 ~ 100까지 숫자중 4의 배수를 더한 결과를 출력해보자!
8. 1 ~ 100까지 숫자를 순회한다.
2 ~ 10 사이의 랜덤한 숫자를 선택하고 이 숫자의 배수를 출력해보도록 한다.
9. 1 ~ 100까지의 숫자를 순회한다.
2 ~ 10 사이의 랜덤한 숫자를 선택하고 이 숫자의 배수를 출력한다.
다음 루프에서 다시 랜덤 숫자를 선택하고 해당 숫자의 배수를 출력한다.
그 다음 루프에서 다시 작업을 반복한다.
끝까지 순회 했을때 출력된 숫자들의 합은 얼마인가 ?
10. 1 ~ 100까지의 숫자를 순회한다.
9번과 유사하게 2 ~ 10을 가지고 작업을 진행한다.
다만 이번에는 배수를 찾는게 아니라 랜덤한 숫자가 나온만큼만 이동하고
이동했을때 나온 숫자들의 합을 계산하도록 만들어보자!
[출처] 문제 은행 [ 2 ] (에디로봇아카데미) | 작성자 링크쌤
🔍풀이
package questionBank.practice2;
import java.util.Random;
public class First {
// 1. 65 ~ 122 사이의 랜덤한 문자를 생성하도록 한다.
// 여기서 소문자나 대문자가 아니라면 다시 생성하도록 프로그램을 만들어보자
public static void main(String[] args) {
Random random = new Random();
while (true){
int num = random.nextInt(65,122);
System.out.println("num : " + num);
if ((65 <= num && num <= 90)
|| (97 <= num && num <= 122)){
char ch = (char)num;
System.out.println("ch : " + ch);
break;
}else {
System.out.println("알파벳 대문자 혹은 소문자가 아닙니다.");
System.out.println();
}
}
}
}
아스키코드 참고 (문자 ↔ 숫자)
https://blog.naver.com/PostView.nhn?blogId=jysaa5&logNo=221831226674
package questionBank.practice2;
import java.util.ArrayList;
public class Second {
// 2. 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ...
// 일명 피보나치 수열의 20번째 항을 구하도록 프로그램 해보자!
private static final int INDEX = 20;
public static void main(String[] args) {
ArrayList<Integer> fibonacci = new ArrayList<>();
int num1 = 0;
int num2 = 1;
int result = 0;
for (int i = 1; i <= INDEX; i++) {
fibonacci.add(result);
System.out.println(i + "번째 result : " + result);
result = num1 + num2;
num1 = num2;
num2 = result;
}
System.out.println();
System.out.println(INDEX + "번째 피보나치 수열 : " + fibonacci.get(INDEX - 1));
}
}
package questionBank.practice2;
public class Third {
// 1, 1, 1, 2, 3, 4, 6, 9, 13, 19, 28, 41, 60, 88, 129, ...
// 이와 같은 숫자의 규칙을 찾아 25번째 항을 구하도록 프로그램 해보자!
private static final int INDEX = 20;
public static void main(String[] args) {
//인덱스가 0부터 시작한다 치면, 인덱스 0 + 2번의 값이 첫 번째 값이 된다.
int[] result = new int[INDEX + 1];
for (int i = 0; i <= INDEX; i++) {
if (result[2] == 0){
result[i] = 1;
} else {
int num = (result[i - 3]) + (result[i - 1]);
result[i] = num;
}
System.out.println("result : " + result[i]);
}
System.out.println();
System.out.println(INDEX + "번째 값 : " + result[INDEX - 1]);
}
}
package questionBank.practice2;
public class Fourth {
// 4. 구구단 7단을 출력해보자!
private static final int DAN = 7;
public static void main(String[] args) {
System.out.println("***구구단 " + DAN +"단!***");
for (int i = 1; i <= 9; i++) {
System.out.println(DAN + " * " + i +" = " + (DAN * i));
}
}
}
package questionBank.practice2;
public class Fifth {
// 5. 1 ~ 100까지 숫자중 짝수만 출력해보자
private static final int MIN = 1;
private static final int MAX = 100;
public static void main(String[] args) {
System.out.println( MIN + "부터 " + MAX + "까지의 숫자중 짝수를 출력합니다.");
for (int i = MIN; i <= MAX; i++) {
if(i % 2 == 0){
System.out.print(i + " ");
}
}
}
}
package questionBank.practice2;
public class Sixth {
//6. 1 ~ 100까지 숫자중 3의 배수만 출력해보자!
private static final int MIN = 1;
private static final int MAX = 100;
private static final int NUM = 3;
public static void main(String[] args) {
System.out.println( MIN + "부터 " + MAX + "까지의 숫자중 " + NUM + "의 배수를 출력합니다.");
for (int i = MIN; i <= MAX; i++) {
if(i % NUM == 0){
System.out.print(i + " ");
}
}
}
}
package questionBank.practice2;
public class Seventh {
// 7. 1 ~ 100까지 숫자중 4의 배수를 더한 결과를 출력해보자!
private static final int MIN = 1;
private static final int MAX = 100;
private static final int NUM = 4;
public static void main(String[] args) {
int sum = 0;
System.out.println( MIN + "부터 " + MAX + "까지의 숫자중 " + NUM + "의 배수의 합을 출력합니다.");
for (int i = MIN; i <= MAX; i++) {
if(i % NUM == 0){
System.out.print(i + " ");
sum = sum + i ;
}
}
System.out.println();
System.out.println("sum = " + sum );
}
}
package questionBank.practice2;
import java.util.ArrayList;
import java.util.Random;
public class Eighth {
// 8. 1 ~ 100까지 숫자를 순회한다.
// 2 ~ 10 사이의 랜덤한 숫자를 선택하고 이 숫자의 배수를 출력해보도록 한다.
private static final int MIN = 1;
private static final int MAX = 100;
public static void main(String[] args) {
allMultiple();
}
/**
* multipleNum메서드에서 랜덤한 숫자 ranNum을 부여 받고,
* number배열에서 ranNum의 배수인 숫자를 ArrayList<Integer> allMultiple에 저장한다.
* @return ArrayList<Integer> allMultiple 값을 반환
*/
public static ArrayList<Integer> allMultiple(){
ArrayList<Integer> allMultiple = new ArrayList<>();
int ranNum = multipleNum(2, 10);
System.out.print(ranNum + "의 배수 : ");
for (int i = MIN; i < allNumber().length; i++) {
if (allNumber()[i] % ranNum == 0){
allMultiple.add(i);
System.out.print(i + " ");
}
}
return allMultiple;
}
/**
* MIN부터 MAX까지의 모든 숫자를 int배열 number에 저장
* @return int배열 number 반환
*/
public static int[] allNumber(){
int[] number = new int[MAX + 1];
for (int i = MIN; i < MAX + 1; i++) {
number[i] = i;
}
return number;
}
/**
* 범위를 입력하면 그 범위 안에서 랜덤한 값을 반환하는 메서드.
* @param min 범위 시작 값
* @param max 범위 마지막 값
* @return 범위 사이의 랜덤한 수 반환
*/
public static int multipleNum(int min, int max){
Random random = new Random();
return random.nextInt(min, max+1);
}
}
package questionBank.practice2;
import java.util.ArrayList;
public class Ninth {
// 9. 1 ~ 100까지의 숫자를 순회한다.
// 2 ~ 10 사이의 랜덤한 숫자를 선택하고 이 숫자의 배수를 출력한다.
// 다음 루프에서 다시 랜덤 숫자를 선택하고 해당 숫자의 배수를 출력한다.
// 그 다음 루프에서 다시 작업을 반복한다.
// 끝까지 순회 했을때 출력된 숫자들의 합은 얼마인가 ?
private static final int loopStopNum = 5;
public static void main(String[] args) {
ArrayList<Integer> loopArr = new ArrayList<>();
int loopSum = 0;
int finalLoopSum = 0;
for (int i = 0; i < loopStopNum; i++) {
loopArr = Eighth.allMultiple();
for (int j = 0; j < loopArr.size(); j++) {
loopSum += loopArr.get(j);
}
System.out.println();
System.out.println("loopSum 합계 : " + loopSum);
finalLoopSum += loopSum;
System.out.println("finalLoopSum 합계 : " + finalLoopSum);
System.out.println();
}
}
}
package questionBank.practice2;
import java.util.ArrayList;
public class Tenth {
// 10. 1 ~ 100까지의 숫자를 순회한다.
// 9번과 유사하게 2 ~ 10을 가지고 작업을 진행한다.
// 다만 이번에는 배수를 찾는게 아니라 랜덤한 숫자가 나온만큼만 이동하고
// 이동했을때 나온 숫자들의 합을 계산하도록 만들어보자!
private static final int loopStopNum = 5;
public static void main(String[] args) {
ArrayList<Integer> nextNumArr = new ArrayList<>();
int nextNumSum = 0;
for (int i = 0 ; i < loopStopNum; i++) {
//nextNumArr이 비워져 있으면 첫 번째 값 저장
if(nextNumArr.isEmpty()){
int ranNum = Eighth.multipleNum(2, 10);
System.out.println("랜덤 숫자 : " + ranNum);
nextNumArr.add(Eighth.allNumber()[ranNum]);
nextNumSum += nextNumArr.get(i);
//아니면 앞 인덱스 값 + 새로운 랜덤 값
// 랜덤한 숫자만큼 이동하기 때문에 기존 값에서 더 해져야함
}else {
int ranNum = Eighth.multipleNum(2, 10);
System.out.println("랜덤 숫자 " + ranNum +"만큼 이동");
nextNumArr.add(nextNumArr.get(i - 1)
+ (Eighth.allNumber()[ranNum]));
nextNumSum += nextNumArr.get(i);
}
}
System.out.println("nextNum = " + nextNumArr);
System.out.println("합계 : " + nextNumSum);
}
}
문제은행[4]
📑문제
*** 초보자용 ***
1. 2 by 2 이중 배열을 초기화해서 아무 값이나 넣어보세요.
2. 1번 문제에서 초기화한 배열을 출력해봅시다.
3. 고양이 클래스를 만들어주세요.
4. 1번 문제를 클래스화 해봅시다.
5. 학생 클래스를 만들어봅시다.
6. 학생 클래스에 수학, 영어, 국어 점수를 입력 받도록 개조합니다.
7. 학생 클래스의 평균을 계산해봅시다.
8. 주사위 클래스를 만들어봅시다.
9. 7번 문제에서 분산을 구해봅시다.
10. 7번 문제에서 표준 편차를 구해봅시다.
*** 챌린지용 ***
1. 여태까지 만들었던 모든 문제들을 클래스화 해봅시다 ^^
[출처] 문제 은행 [ 4 ] (에디로봇아카데미) | 작성자 링크쌤
🔍풀이
Cat
package questionBank.practice4;
import java.util.Arrays;
public class Cat {
public static void main(String[] args) {
String[][] catInfo = {{"누리", "로또", "동글이"},{"2세", "4세", "3세"}};
System.out.println("고양이 정보 : " + Arrays.deepToString(catInfo));
}
}
Students
package questionBank.practice4;
import jdk.swing.interop.SwingInterOpUtils;
import java.util.Scanner;
public class Students {
static StudentsScore studentsAve = new StudentsScore();
public static void main(String[] args) {
subjectScore();
}
/**
* 과목 점수를 입력하면 평균점수/분산/표준편차를 출력해주는 메서드
*/
public static void subjectScore(){
Scanner sc = new Scanner(System.in);
System.out.print("수학 점수 : ");
int mathScore = sc.nextInt();
System.out.print("영어 점수 : ");
int engScore = sc.nextInt();
System.out.print("국어 점수 : ");
int korScore = sc.nextInt();
System.out.println();
System.out.println("과목 평균 점수 : " +
studentsAve.ave(mathScore, engScore, korScore));
System.out.println("분산 : " +
studentsAve.variance(mathScore, engScore, korScore));
System.out.println("표준편차 : " +
studentsAve.standardDeviation(mathScore, engScore, korScore));
}
}
StudentsScore
package questionBank.practice4;
public class StudentsScore {
private int mathScore;
private int engScore;
private int korScore;
public StudentsScore() {
}
/**
* 입력 받은 과목 점수의 평균을 반환하는 메서드
* @param mathScore 수학 점수
* @param engScore 영어 점수
* @param korScore 국어 점수
* @return 85.33333333333333
*/
public double ave(int mathScore, int engScore, int korScore) {
this.mathScore = mathScore;
this.engScore = engScore;
this.korScore = korScore;
return (double) (mathScore + engScore + korScore) / 3;
}
/**
* 입력 받은 과목 점수의 분산 값을 반환하는 메서드
* @param mathScore 수학 점수
* @param engScore 영어 점수
* @param korScore 국어 점수
* @return
*/
public double variance(int mathScore, int engScore, int korScore) {
this.mathScore = mathScore;
this.engScore = engScore;
this.korScore = korScore;
double ave = ave(mathScore, engScore, korScore);
//과목 별 편차 구하기
mathScore = (int) Math.pow(mathScore-ave, 2);
engScore = (int) Math.pow(engScore-ave, 2);
korScore = (int) Math.pow(korScore-ave, 2);
return (mathScore + engScore + korScore) / 3;
}
/**
* 력 받은 과목 점수의 표준편차 값을 반환 하는 메서드
* @param mathScore 수학 점수
* @param engScore 영어 점수
* @param korScore 국어 점수
* @return
*/
public double standardDeviation(int mathScore, int engScore, int korScore) {
this.mathScore = mathScore;
this.engScore = engScore;
this.korScore = korScore;
return Math.pow(variance(mathScore, engScore, korScore), 2);
}
}
'Backend > JAVA' 카테고리의 다른 글
[JAVA] JAVA 설계시 패키지 구분 (entity/utillity/controller) (0) | 2022.08.03 |
---|---|
[JAVA] 특강 20일차 : 코딩 훈련! 문제 풀이 (상속&인터페이스&추상&다형성&파일) (0) | 2022.07.19 |
[JAVA] 특강 19일차 : 코딩 훈련! 문제 풀이 (③ 상속&인터페이스&추상&다형성) (0) | 2022.07.15 |
[JAVA] 특강 19일차 : 코딩 훈련! 문제 풀이 (② 상속) (0) | 2022.07.15 |
[JAVA] 특강 19일차 : 코딩 훈련! 문제 풀이 (① 상속) (0) | 2022.07.15 |